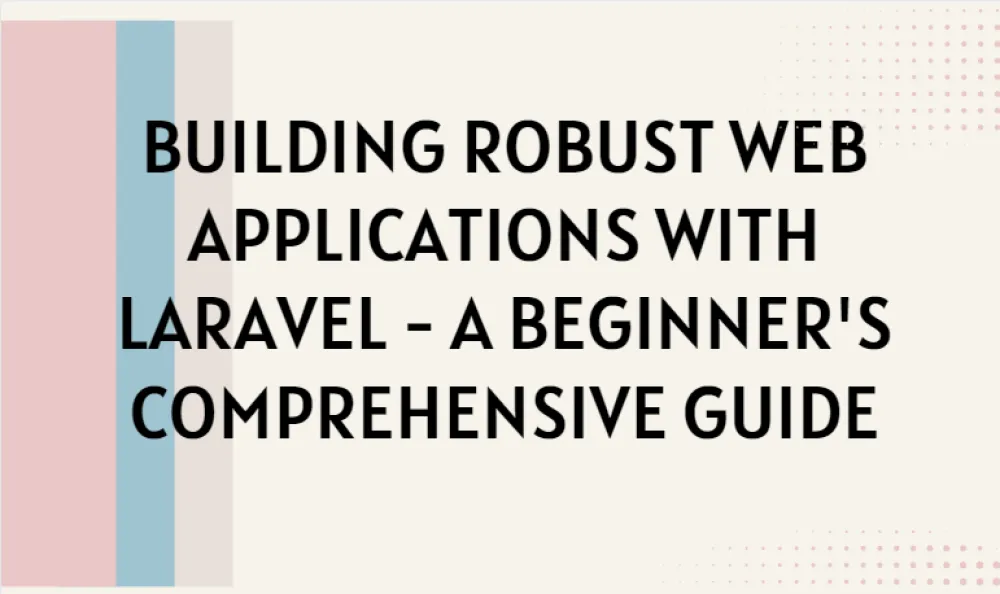
Building Robust Web Applications with Laravel - A Beginner's Comprehensive Guide
Are you ready to embark on the journey of building robust web applications? Laravel, one of the most popular PHP frameworks, provides the tools and features you need to create powerful and scalable web applications with ease. In this comprehensive beginner's guide, we'll walk you through the process of building robust web applications using Laravel, step by step, with beginner-friendly examples.
Step 1: Setting Up Your Laravel Environment
The first step in building a web application with Laravel is setting up your development environment. Learn how to install Laravel using Composer, create a new Laravel project, and configure your development environment for smooth development.
composer create-project --prefer-dist laravel/laravel mywebapp
Step 2: Understanding Laravel's MVC Architecture
Laravel follows the Model-View-Controller (MVC) architectural pattern, which provides a structured way to organize your codebase. Understand the roles of models, views, and controllers in Laravel and how they interact to build web applications.
// Example of a Laravel controller
namespace App\Http\Controllers;
use App\Models\User;
class UserController extends Controller
{
public function index()
{
$users = User::all();
return view('users.index', ['users' => $users]);
}
}
Step 3: Creating Routes and Views
Routes define the entry points for your application, while views handle the presentation logic. Learn how to define routes in Laravel's routes/web.php
file and create views using Blade templating engine to render HTML content.
// Example of defining a route
Route::get('/users', 'UserController@index');
// Example of a Blade view
@extends('layouts.app')
@section('content')
<h1>Users</h1>
<ul>
@foreach ($users as $user)
<li>{{ $user->name }}</li>
@endforeach
</ul>
@endsection
Step 4: Database Interactions with Eloquent ORM
Laravel's Eloquent ORM simplifies database interactions by providing a fluent and expressive interface for working with database tables. Learn how to define models, create migrations, and perform common database operations like querying and updating data.
// Example of an Eloquent model
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
// Model properties and methods
}
Step 5: Implementing Authentication and Authorization
Security is crucial for web applications. Learn how to implement user authentication and authorization in Laravel using Laravel's built-in authentication scaffolding and middleware.
php artisan make:auth
Step 6: Testing and Deployment
Thoroughly test your Laravel application to ensure it functions as expected and is free from bugs. Learn how to write unit tests and feature tests using Laravel's testing utilities and PHPUnit. Finally, deploy your Laravel application to a web server for production use.
By following these steps and examples, beginners can build robust web applications using Laravel and unleash their creativity in developing innovative solutions.