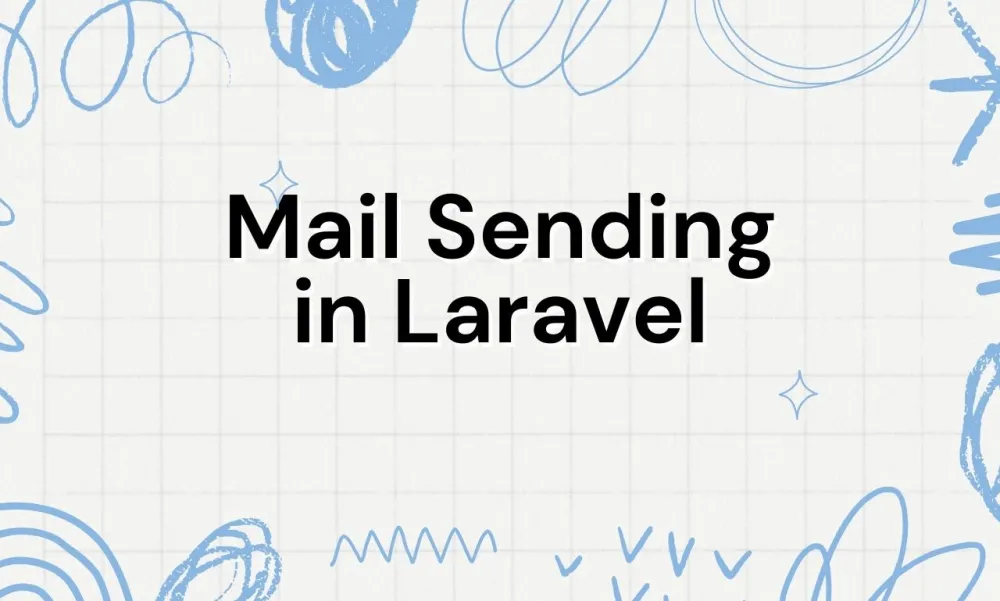
Mail Sending in Laravel
In the realm of web development, efficient and reliable email communication is indispensable. Whether it's sending notifications, newsletters, password resets, or transactional emails, having a robust system in place is crucial. Laravel, the popular PHP framework, simplifies this task with its built-in email sending capabilities, offering developers a seamless way to integrate email functionality into their applications.
Laravel provides a powerful and expressive API for sending emails through multiple drivers such as SMTP, Mailgun, mailtrap, and more. This flexibility allows developers to choose the most suitable email service provider or configure their own email server according to project requirements. We are going to use mailtrap here.
Open. env file and past the mailtrap smtp credential its look like.
MAIL_MAILER=smtp
MAIL_HOST=sandbox.smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=<your mailtrap username>
MAIL_PASSWORD=<your mailtrap password>
MAIL_ENCRYPTION=null
MAIL_FROM_ADDRESS="<from address here> "
MAIL_FROM_NAME="${APP_NAME}"
Let’s create two new routes:
Route::get('mail-send', [MailSendController::class, 'index'])->name('mail-send-page');
Route::post('mail-send', [MailSendController::class, 'send'])->name('mail-send');
Here
mail-send-page is a simple html and bootstrap page where have some input field
like name, email, phone, message. When click submit button we will take all
information and send to mail. mail-send route is a post route using this route
acutely we are going send mail.
MailSendController controller is used for handle those above route lets create a controller with the help of laravel artisan command.
php artisan make:controller MailSendController
Past the given
code inside controller
<?php namespace App\Http\Controllers; use App\Mail\MailSendExample; use Illuminate\Contracts\View\View; use Illuminate\Http\RedirectResponse; use Illuminate\Http\Request; use Illuminate\Support\Facades\Mail; class MailSendController extends Controller { public function index() : View { return view('mail-send-example.index'); } public function send(Request $request) : RedirectResponse { // please make validation before next line which is best prectices $data = [ 'name' => $request->name, 'email' => $request->email, 'phone' => $request->phone, 'message' => $request->message ]; Mail::to('rasel.laravel@gmail.com') ->send(new MailSendExample($data)); return redirect()->back()->with('success','Mail has been sent successfully'); } }
Create a view file to show our form inside resources/views/mail-send-example/index.blade.php and past this bellow code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Laravel Mail Send Example</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container">
@if(session()->has('success'))
<div class="alert alert-success">{{ session()->get('success') }}</div>
@endif
<form action="{{ route('mail-send') }}" method="post">
@csrf
<div class="row">
<div class="col-md-6 mb-3">
<label for="name" class="form-label">Name</label>
<input type="text" class="form-control" id="name" name="name">
</div>
<div class="col-md-6 mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" name="email">
</div>
<div class="col-md-6 mb-3">
<label for="phone" class="form-label">Phone</label>
<input type="text" class="form-control" id="phone" name="phone">
</div>
<div class="col-md-12 mb-3">
<label for="message" class="form-label">Message</label>
<textarea class="form-control" id="message" name="message"></textarea>
</div>
<button type="submit" class="btn btn-info">Send</button>
</div>
</form>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.min.js"></script>
</body>
</html>
Now let’s create a mailable where we get laraval mail template with the help of artisan command
php artisan make:mail MailSendExample –markdown
remember this commend will generate mailable along with mail template which located resources/views/mail/mail-send-example
Open App/Mail/MailSendExample and past bellow code.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
class MailSendExample extends Mailable
{
use Queueable, SerializesModels;
public function __construct(public array $data){}
public function envelope(): Envelope
{
return new Envelope(
subject: 'Mail Send Example Subject',
);
}
public function content(): Content
{
return new Content(
markdown: 'mail.mail-send-example',
);
}
public function attachments(): array
{
return [];
}
}
go to resourecs/views/mail/ mail-send-example and past this code
<x-mail::message> # Introduction of you email Name: {{ $data['name'] }} <br> Email: {{ $data['email'] }}<br> Phone: {{ $data['phone'] }}<br> Message: {{ $data['message'] }}<br> <x-mail::button :url="''"> Visit Website </x-mail::button> Thanks,<br> {{ config('app.name') }} </x-mail::message>
Testing: php artisan serve
htttp://localhost:8000/mail-send and fill up form and submit mail will be send to your mailtrap inbox.
Read More about mail sending:
How to send mail using laravel queue
Happy Coding.