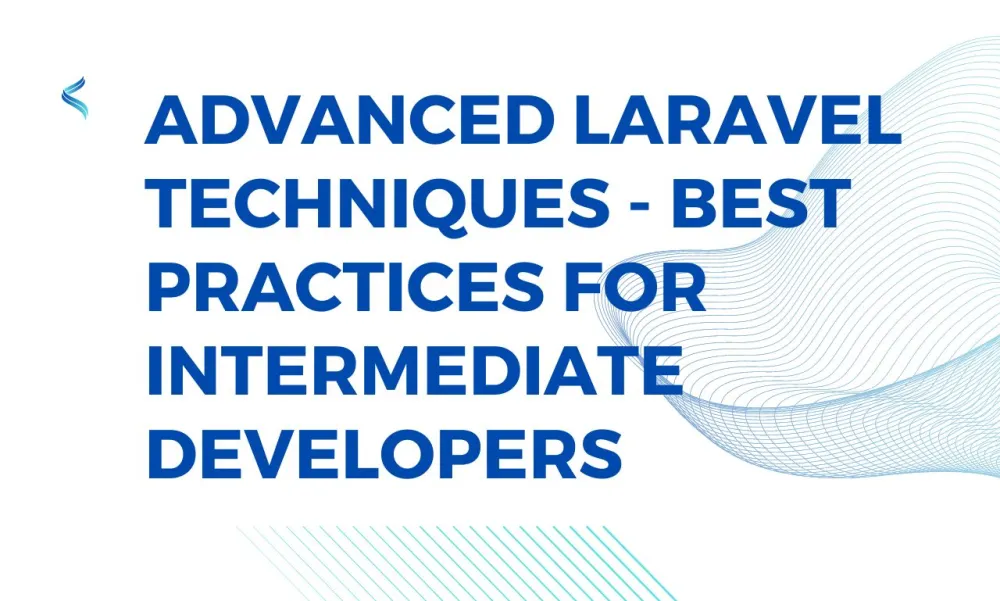
Advanced Laravel Techniques - Best Practices for Intermediate Developers
Ready to level up your Laravel skills and dive into more advanced techniques? As an intermediate Laravel developer, you've already mastered the basics and built some impressive projects. Now it's time to explore advanced techniques and best practices to take your Laravel development expertise to new heights. In this guide, we'll walk you through advanced techniques and best practices in Laravel, step by step, with beginner-friendly examples.
Step 1: Dependency Injection and Service Providers
Laravel's dependency injection container allows you to manage class dependencies effortlessly. Learn how to leverage dependency injection and create custom service providers to bind dependencies and organize your application's services efficiently.
// Example of dependency injection in a controller
use App\Services\PaymentService;
class OrderController extends Controller
{
protected $paymentService;
public function __construct(PaymentService $paymentService)
{
$this->paymentService = $paymentService;
}
}
Step 2: Use Middleware for Cross-Cutting Concerns
Middleware provides a convenient mechanism for filtering HTTP requests entering your application. Explore advanced middleware techniques such as route middleware, global middleware, and middleware groups to handle cross-cutting concerns like authentication, authorization, and request validation.
// Example of custom middleware
namespace App\Http\Middleware;
use Closure;
class RedirectIfNotAdmin
{
public function handle($request, Closure $next)
{
if (! $request->user()->isAdmin()) {
return redirect('home');
}
return $next($request);
}
}
Step 3: Harness the Power of Queues and Jobs
Laravel's queue system allows you to defer the processing of time-consuming tasks, improving application performance and responsiveness. Learn how to create and dispatch jobs, configure queue connections, and monitor queue execution to optimize your application's workload.
// Example of dispatching a job
use App\Jobs\ProcessOrder;
ProcessOrder::dispatch($order);
Step 4: Optimize Database Performance with Eloquent Tips
Eloquent ORM provides powerful features for interacting with your database. Discover advanced Eloquent techniques such as eager loading, query scopes, and database transactions to optimize database performance and write efficient database queries.
// Example of eager loading relationships
$posts = App\Post::with('comments')->get();
Step 5: Implement API Authentication and Rate Limiting
Secure your API endpoints and prevent abuse by implementing authentication and rate limiting. Explore Laravel Passport for API authentication and Laravel's built-in rate limiting middleware to protect your API routes from abuse and unauthorized access.
// Example of API authentication using Laravel Passport
Route::post('/oauth/token', '\Laravel\Passport\Http\Controllers\AccessTokenController@issueToken');
Route::middleware('auth:api')->get('/user', function (Request $request) {
return $request->user();
});
Step 6: Test Driven Development (TDD) with Laravel
Embrace Test-Driven Development (TDD) to write robust and reliable Laravel applications. Learn how to write unit tests and feature tests using Laravel's testing utilities and PHPUnit to ensure your application functions as expected and prevent regressions.
By mastering these advanced techniques and best practices, intermediate Laravel developers can elevate their skills and build more sophisticated and scalable applications.